Mobile apps often need to communicate with remote servers to fetch data, send updates, or provide real-time information. Networking and web APIs are crucial for enabling these interactions. This guide will explore the essentials of networking and web APIs in Android development, emphasizing the role of custom Android app development services in creating seamless and efficient app experiences.
1. Understanding Networking in Android
– Importance of Networking: Explain why networking is essential in modern Android apps.
– Common Use Cases: Discuss scenarios where networking is used, such as fetching data from a server, uploading files, and real-time communication.
Importance of Networking
Networking allows your app to access and interact with remote resources. This interaction is vital for:
– Fetching Data: Retrieve data from web servers to display in the app.
– Real-time Updates: Keep the app updated with live information.
– Synchronization: Sync data across multiple devices and platforms.
Common Use Cases
Understanding the use cases helps in designing effective networking solutions:
– RESTful APIs: Communicate with servers using RESTful APIs to perform CRUD operations (Create, Read, Update, Delete).
– GraphQL APIs: Use GraphQL for more flexible and efficient data querying.
– WebSockets: Implement WebSockets for real-time communication and updates.
2. Choosing the Right Networking Library
– Overview of Popular Libraries: Introduce popular networking libraries like Retrofit, OkHttp, and Volley.
– Criteria for Selection: Discuss criteria for selecting a networking library based on project requirements.
Popular Networking Libraries
Several networking libraries can simplify the implementation process:
– Retrofit: A type-safe HTTP client for Android and Java.
– OkHttp: An efficient HTTP & HTTP/2 client.
– Volley: A powerful library for handling network operations, particularly suited for smaller requests and responses.
Criteria for Selection
Selecting the right library depends on various factors:
– Project Requirements: Assess the complexity and specific needs of your project.
– Ease of Use: Consider how easy it is to implement and maintain the library.
– Community Support: Evaluate the library’s community support and documentation.
3. Setting Up Retrofit for Networking
– Introduction to Retrofit: Explain what Retrofit is and why it’s widely used.
– Integration: Steps to integrate Retrofit into an Android project.
– Basic Usage: Provide examples of making GET and POST requests.
Introduction to Retrofit
Retrofit is a type-safe HTTP client for Android and Java, developed by Square. It simplifies the process of making network requests by turning your HTTP API into a Java interface.
Integration
To integrate Retrofit into your Android project:
– Dependencies: Add the necessary dependencies to your `build.gradle` file:
“`groovy
implementation ‘com.squareup.retrofit2:retrofit:2.9.0’
implementation ‘com.squareup.retrofit2:converter-gson:2.9.0’
“`
– Interface Definition: Define your API endpoints using an interface:
“`java
public interface ApiService {
@GET(“users/{user}/repos”)
Call<List<Repo>> listRepos(@Path(“user”) String user);
}
“`
– Retrofit Instance: Create a Retrofit instance:
“`java
Retrofit retrofit = new Retrofit.Builder()
.baseUrl(“https://api.github.com/”)
.addConverterFactory(GsonConverterFactory.create())
.build();
ApiService service = retrofit.create(ApiService.class);
“`
Basic Usage
Making network requests with Retrofit is straightforward:
– GET Request: Fetch data from the server:
“`java
Call<List<Repo>> repos = service.listRepos(“octocat”);
repos.enqueue(new Callback<List<Repo>>() {
@Override
public void onResponse(Call<List<Repo>> call, Response<List<Repo>> response) {
if (response.isSuccessful()) {
List<Repo> repos = response.body();
// Handle the response
}
}
@Override
public void onFailure(Call<List<Repo>> call, Throwable t) {
// Handle the error
}
});
“`
– POST Request: Send data to the server:
“`java
@POST(“users/new”)
Call<User> createUser(@Body User user);
“`
4. Handling Responses and Errors
– Response Handling: Explain how to handle successful responses.
– Error Handling: Strategies for handling errors and exceptions.
Response Handling
Handling responses correctly ensures a smooth user experience:
– Success: Process the response body when the request is successful.
“`java
@Override
public void onResponse(Call<List<Repo>> call, Response<List<Repo>> response) {
if (response.isSuccessful()) {
List<Repo> repos = response.body();
// Update the UI or process the data
}
}
“`
– Error: Handle error scenarios gracefully.
“`java
@Override
public void onFailure(Call<List<Repo>> call, Throwable t) {
// Show an error message or take appropriate action
}
“`
Error Handling
Errors are inevitable, so it’s essential to handle them effectively:
– Network Errors: Detect and manage network-related issues like timeouts and connectivity problems.
– Server Errors: Handle HTTP error codes (e.g., 404, 500) appropriately.
– User-Friendly Messages: Display meaningful error messages to the user.
5. Working with JSON and Data Parsing
– Parsing JSON: Techniques for parsing JSON responses.
– Libraries for Parsing: Introduction to libraries like Gson and Moshi.
Parsing JSON
Parsing JSON data is a common task in Android development:
– Manual Parsing: Use native JSON parsing methods like `JSONObject` and `JSONArray`.
– Automatic Parsing: Utilize libraries to simplify the parsing process.
Libraries for Parsing
Using libraries can make JSON parsing more efficient:
– Gson: A popular library for converting JSON to Java objects.
“`java
Gson gson = new Gson();
User user = gson.fromJson(jsonString, User.class);
“`
– Moshi: Another powerful library for JSON parsing and serialization.
“`java
Moshi moshi = new Moshi.Builder().build();
JsonAdapter<User> jsonAdapter = moshi.adapter(User.class);
User user = jsonAdapter.fromJson(jsonString);
“`
6. Managing API Calls Efficiently
– Concurrency Handling: Strategies for handling multiple API calls concurrently.
– Caching: Implementing caching to improve performance and reduce network load.
Concurrency Handling
Managing multiple API calls efficiently can improve performance:
– AsyncTask: Use AsyncTask for simple asynchronous operations (deprecated in newer Android versions).
– Executors: Utilize ExecutorService for more complex concurrency handling.
– Coroutines: Leverage Kotlin coroutines for modern, efficient concurrency management.
“`kotlin
CoroutineScope(Dispatchers.IO).launch {
val response = service.listRepos(“octocat”).await()
withContext(Dispatchers.Main) {
if (response.isSuccessful) {
val repos = response.body()
// Update the UI
}
}
}
“`
Caching
Caching can significantly improve performance and reduce network load:
– HTTP Caching: Use HTTP cache headers to control caching behavior.
– Local Caching: Store frequently accessed data locally using Room or SQLite.
“`java
@Entity
public class User {
@PrimaryKey
public int id;
public String name;
}
“`
7. Securing Network Communication
– HTTPS and SSL: Importance of using HTTPS and SSL certificates.
– Authentication: Implementing secure authentication methods like OAuth.
HTTPS and SSL
Securing network communication is critical:
– HTTPS: Always use HTTPS to encrypt data in transit.
– SSL Pinning: Implement SSL pinning to prevent man-in-the-middle attacks.
Authentication
Secure authentication ensures only authorized users can access your API:
– Basic Authentication: Simple but less secure.
– OAuth: Use OAuth for a more secure and scalable authentication solution.
“`java
@Override
public void apply(Request request, Request.Builder builder) {
builder.addHeader(“Authorization”, “Bearer ” + accessToken);
}
“`
8. Handling Different Network Conditions
– Network Connectivity: Techniques for checking network status.
– Offline Support: Implementing offline support for your app.
Network Connectivity
Monitoring network connectivity ensures your app behaves correctly under various conditions:
– Connectivity Manager: Use ConnectivityManager to check network status.
“`java
ConnectivityManager cm = (ConnectivityManager) getSystemService(Context.CONNECTIVITY_SERVICE);
NetworkInfo activeNetwork = cm.getActiveNetworkInfo();
boolean isConnected = activeNetwork != null && activeNetwork.isConnectedOrConnecting();
“`
Offline Support
Providing offline support enhances user experience:
– Local Storage: Save data locally using SQLite or Room.
– Sync Strategies: Implement sync strategies to update local data when the network is available.
9. Best Practices for Networking in Android
– Efficient Data Loading: Techniques for efficient data loading and background tasks.
– Error Reporting: Implementing robust error reporting mechanisms.
Efficient
Data Loading
Efficient data loading improves performance and user experience:
– Lazy Loading: Load data on demand rather than all at once.
– Pagination: Implement pagination to handle large datasets efficiently.
Error Reporting
Robust error reporting helps in debugging and improving the app:
– Crashlytics: Use Firebase Crashlytics for detailed error reporting.
– Custom Error Handling: Implement custom error handling to capture and log errors.
Final Words
Networking and web APIs are fundamental components of modern Android apps. By understanding and implementing best practices, you can create robust, efficient, and secure applications. Custom app developers can provide expertise and support to ensure your app leverages networking capabilities to their fullest potential.
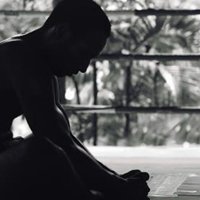
Daniel J. Morgan is the founder of Invidiata Magazine, a premier publication showcasing luxury living, arts, and culture. With a passion for excellence, Daniel has established the magazine as a beacon of sophistication and refinement, captivating discerning audiences worldwide.