In the intricate world of Java programming, encountering syntax errors is akin to stumbling upon hidden landmines that threaten to derail your projects. These errors, often simple yet elusive, can transform a promising application into a frustrating marathon of debugging sessions.
Fortunately, this blog is designed to arm you with essential strategies to detect, rectify, and preempt these syntax pitfalls.
By mastering these skills, you can ensure that your Java applications run seamlessly and efficiently, sparing you unnecessary headaches and boosting your coding productivity.
The Significance of Correct Syntax in Java Programming
The significance of correct syntax in Java programming cannot be overstated. Java’s syntax rules form the very foundation of its functionality, where every semicolon, bracket, and keyword plays a crucial role in directing how the Java compiler interprets and executes code. A single misplaced character can halt an entire application, akin to the chaos caused by incorrectly placed punctuation in a book both confusing and ineffective.
Spotting and fixing them requires careful attention to code structure and error messages provided by the compiler, which often point to the exact location of the issue. Staying alert to such mistakes is especially important when working on projects remotely, such as during travel in Thailand. Developers can stay connected and manage code fixes efficiently using e sim thailand for seamless communication and access to resources.
Among these tools, Java is celebrated for its versatility and broad application, yet demanding meticulous adherence to syntax rules, illustrating the interconnectedness of modern technology.
Common Types of Syntax Errors in Java
1. Missing Semicolons: The Silent Killers
- Incorrect: int x = 5 System.out.println(x);
- Correct: int x = 5; System.out.println(x);
Semicolons are crucial for terminating statements.
2. Mismatched Braces: The Bracket Breakdown
Incorrect
public class Example {
if (true) {
System. out.println(“This is correct”);
// Missing closing brace
}
Correct
public class Example {
if (true) {
System. out.println(“This is correct”);
}
}
Curly braces {} define blocks of code; ensure each opening brace has a matching closing brace.
3. Incorrect Method Signatures: The Function Faux Pas
- Incorrect: public myMethod() { return “Hello”; }
- Correct: public String myMethod() { return “Hello”; }
Method signatures must include return types and correct naming.
4. Case Sensitivity Blunders: The Capital Offense
- Incorrect: String myString = “Hello”; system.out.println(mystring);
- Correct: String myString = “Hello”; System.out.println(myString);
Java is case-sensitive; pay attention to the case of identifiers.
5. Uninitialized Variables: The Null Pointer Nightmares
- Incorrect: int x; System.out.println(x);
- Correct: int x = 0; System.out.println(x);
Always initialize variables to avoid compilation errors and runtime exceptions.
Understanding and correcting these frequent syntax errors will improve your Java coding effectiveness.
Strategies for Identifying Syntax Errors
1. Use IDE Tools: Implement IDE features like real-time error highlighting, quick-fix suggestions, and code completion to prevent and correct errors efficiently.
2. Decode Compiler Messages: Pay attention to compiler error messages that detail the exact location and nature of errors, such as missing semicolons.
3. Conduct Manual Reviews: Regularly read your code aloud, maintain proper indentation, and check for common mistakes to catch errors manually.
4. Engage in Peer Reviews: Benefit from the fresh perspectives of colleagues and share best coding practices to enhance code quality and reduce errors.
Step-by-Step Guide to Resolving Syntax Errors
Here’s a streamlined approach to fixing syntax errors in Java, organized into simple steps for clarity and ease of understanding:
1. Analyze the Error Message
- Read the Error: Carefully analyze the compiler’s error message which guides you to the problem.
- Locate the Error: Note the file name, line number, and character position indicated.
- Understand the Error Type: Recognize whether it’s a simple error (like a missing semicolon) or something more complex (like a method not found).
2. Navigate to the Error Location
- Use IDE Navigation: Use features in your IDE to quickly jump to the problematic line.
3. Examine Surrounding Code
- Check Adjacent Lines: Errors might be influenced by nearby code. Review lines before and after the error for potential causes.
4. Apply the Appropriate Fix
- For Missing Semicolons: Add them at the end of the indicated statements.
- For Mismatched Braces: Ensure every opening brace has a closing match.
- For Method Signature Errors: Verify the method name, parameters, and return type are correct.
5. Recompile and Verify
- Test the Fixes: Recompile your code to check that the error is resolved and that no new issues have emerged.
6. Repeat for Multiple Errors
- Address Additional Errors: If there are more errors, apply the same steps to each, starting with the first error reported.
Example: Fixing a Common Syntax Error
Original Error
- Example.java:3: error: ‘;’ expected int x = 5 ^
- Example.java:4: error: ‘;’ expected System. out.println(“The value of x is: ” + x) ^
Step-by-Step Fix
- Navigate to line 3, add a semicolon at the end: int x = 5;
- Move to line 4, and add another semicolon: System. out.println(“The value of x is: ” + x);
- Recompile to ensure the fixes are correct.
Corrected Code
java
Copy code
public class Example {
public static void main(String[] args) {
int x = 5;
System. out.println(“The value of x is: ” + x);
}
}
This systematic method ensures effective resolution of syntax errors, enhancing your Java code’s functionality and reliability.
Advanced Tools and Practices to Prevent Syntax Errors
To enhance the quality of Java code and prevent syntax errors, integrating advanced tools and practices into your development process is essential. Static code analysis tools like SonarQube, FindBugs, and PMD can scrutinize your code without execution, pinpointing potential errors and vulnerabilities before they become problematic.
Additionally, IDE plugins such as CheckStyle, SpotBugs, and Error Prone extend error detection capabilities, ensuring common mistakes are caught early. Employing consistent code formatting tools like Google Java Format and Prettier also aids in maintaining clean, error-free code.
Moreover, leveraging version control best practices with Git, incorporating pre-commit hooks, and enforcing code review workflows alongside continuous integration tools like Jenkins and Travis CI can automate syntax checks and enhance code quality. Lastly, fostering a collaborative environment with regular peer reviews and pair programming can prevent many common syntax errors, making your Java projects more robust and reliable.
Impact of Unresolved Syntax Errors on Java Applications
While identifying and fixing syntax errors is crucial, the broader implications of leaving these errors unresolved can have significant consequences. Unresolved syntax errors can lead to security vulnerabilities, such as incomplete error handling that leaves applications open to attacks, or unintended code execution that might expose sensitive data.
Performance may suffer due to inefficient code execution and memory leaks causing gradual degradation. From a maintenance perspective, unresolved errors reduce code readability and increase technical debt, complicating future updates and making maintenance arduous. For end-users, these errors can result in application crashes and incorrect functionality, severely degrading the user experience.
Additionally, the development process itself suffers as more time spent on debugging leads to delays in new features and product releases. Together, these factors underscore the importance of addressing syntax errors promptly to maintain the integrity and efficiency of software development.
Final Thoughts
Effectively managing syntax errors in Java is crucial for robust software development. By utilizing IDE tools, understanding compiler messages, and maintaining diligent code reviews, developers can greatly reduce syntax errors, enhance code quality, and streamline their development process for more reliable and efficient applications.
FAQs
How to resolve syntax errors in Java?
Read the compiler’s error messages for clues, navigate to the indicated line, and make the necessary corrections based on the error type.
How do you fix a syntax error?
Fix syntax errors by using IDE tools for error detection, applying manual code reviews, and ensuring proper code syntax and structure.
How to spot syntax errors?
Spot syntax errors by leveraging IDEs for real-time error highlighting, examining compiler error messages, and conducting thorough code reviews.
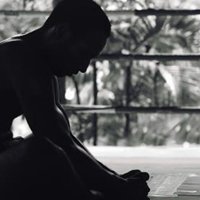
Daniel J. Morgan is the founder of Invidiata Magazine, a premier publication showcasing luxury living, arts, and culture. With a passion for excellence, Daniel has established the magazine as a beacon of sophistication and refinement, captivating discerning audiences worldwide.